SOFTWARE ENGINEERING
[PENDING] BDD on RoR (With RSpec, Capybara and Selenium)

If you are reading this article I assume you already familiar with Test Driven Development or if not, is okay as long as you are still curious, we will get through this.
In test-driven development, each new feature begins with writing a test. —Kent Beck, on Test-Driven Development by Example.
So in TDD, we develop a feature of software based on the test that we have written and Behaviour Driven Development (BDD) built on top the concept of TDD.
Behavior-Driven Development can be understood as writing a test based on a user story.
Here’s an example of BDD:
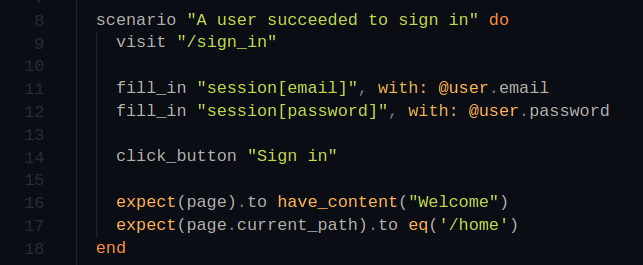
Above, I write a scenario of a user doing sign in and at line 14, when sign_in button gets clicked, the user expects to see a component from the homepage, such as the word “Welcome”, and the route path is expected to be at “/home”.
The syntax from Capybara and RSpec makes the code easy to understand even by a non-developer.
Now enough of the introduction. Let’s start to do some practice.
I will break down the explanation one by one to help you understand each use of RSpec, Capybara, and Selenium.
RSpec
RSpec is a ‘Domain Specific Language’ tool that most frequently used for testing Ruby application.
To start the practice, you can use your existing project or create the new one — even tho I recommend you to start implement BDD on a new project — by run:
$ rails new app
Then, add ‘rspec-rails’ on your Gemfile.
group :development, :test do gem 'rspec-rails', '~> 3.8' end
Don’t forget to run bundle install after adding gems on your Gemfile. Then run:
rails generate rspec:install
It will generate some files.
create .rspec create spec create spec/spec_helper.rb create spec/rails_helper.rb
At the top of your spec/spec_helper.rb add:

spec/spec_helpers.rb
And now rspec set up is ready, you can create a new file inside spec/ folder and write a simple test like this:
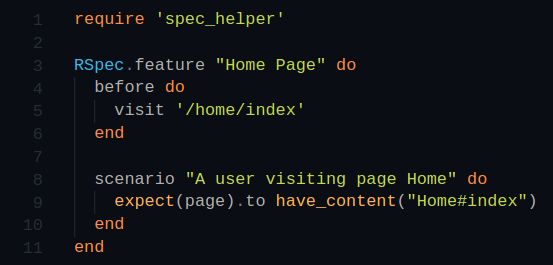
spec/features/home_spec.rb
Try to run:
$ rspec
You will get an error.

Cause, of course, we haven’t created the page yet. Remember TDD, always writing the test first.
To make this practice easier we can generate the ‘/home/index’ page by run:
$ rails generate controller Home index
then run
$rspec
Voila, the test succeeded.
Capybara
Like RSpec, Capybara is a DSL tool that can simulate test application. Capybara works by locating relevant elements in the DOM (Document Object Model) and execute action such as click button, find a link, etc.
Now let’s add a new scenario
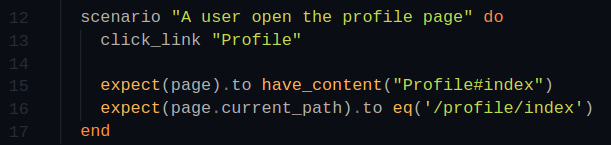
This scenario expects to find a link that we haven’t created yet. So we need to add the element at our home/index file:

app/views/home/index.html.erb
And then generate the profile page by run:
$ rails generate controller Profile index
try to run
$ rspec
Now, we have finished applying a simple test using Capybara. By default, Capybara will only locate visible elements. You could find more information about Capybara by reading the documentation.
Selenium
Selenium is an automation framework that can be used as a driver for Capybara. It is capable to operate a web browser like resizing the browser window, moving the pointer to hover some element as if a person operating it.
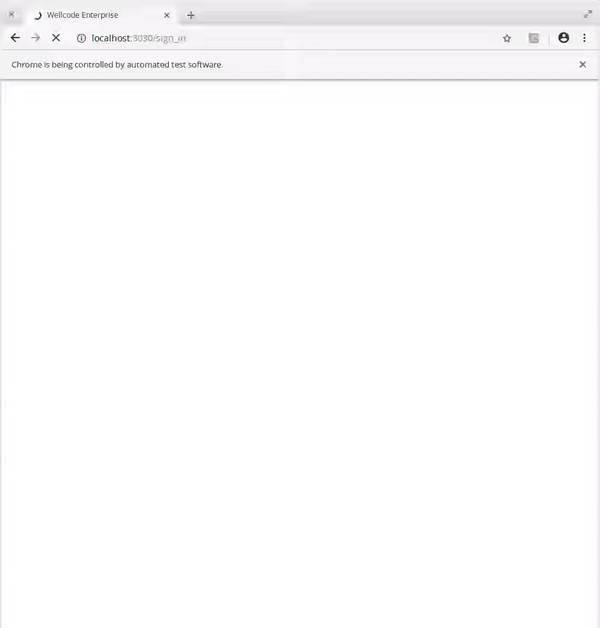
Above, you could see the example of some tests runs using Selenium. By default, rails already install ‘selenium-webdriver’ gem. So what we need to do just add one line of code inside spec/spec_helper.rb:

spec/spec_helper.rb
Try to run:
$rspec
Now you can see a chrome window opens and doing the test automatically.
You can also use :selenium_chrome_headless to make the chrome window run your test without being seen.
Now all the set up is ready. There are still many things to learn. I hope this article could help you understand the use of RSpec, Capybara, and Selenium in implementing your BDD test.