Get to Know Progressive Web Apps
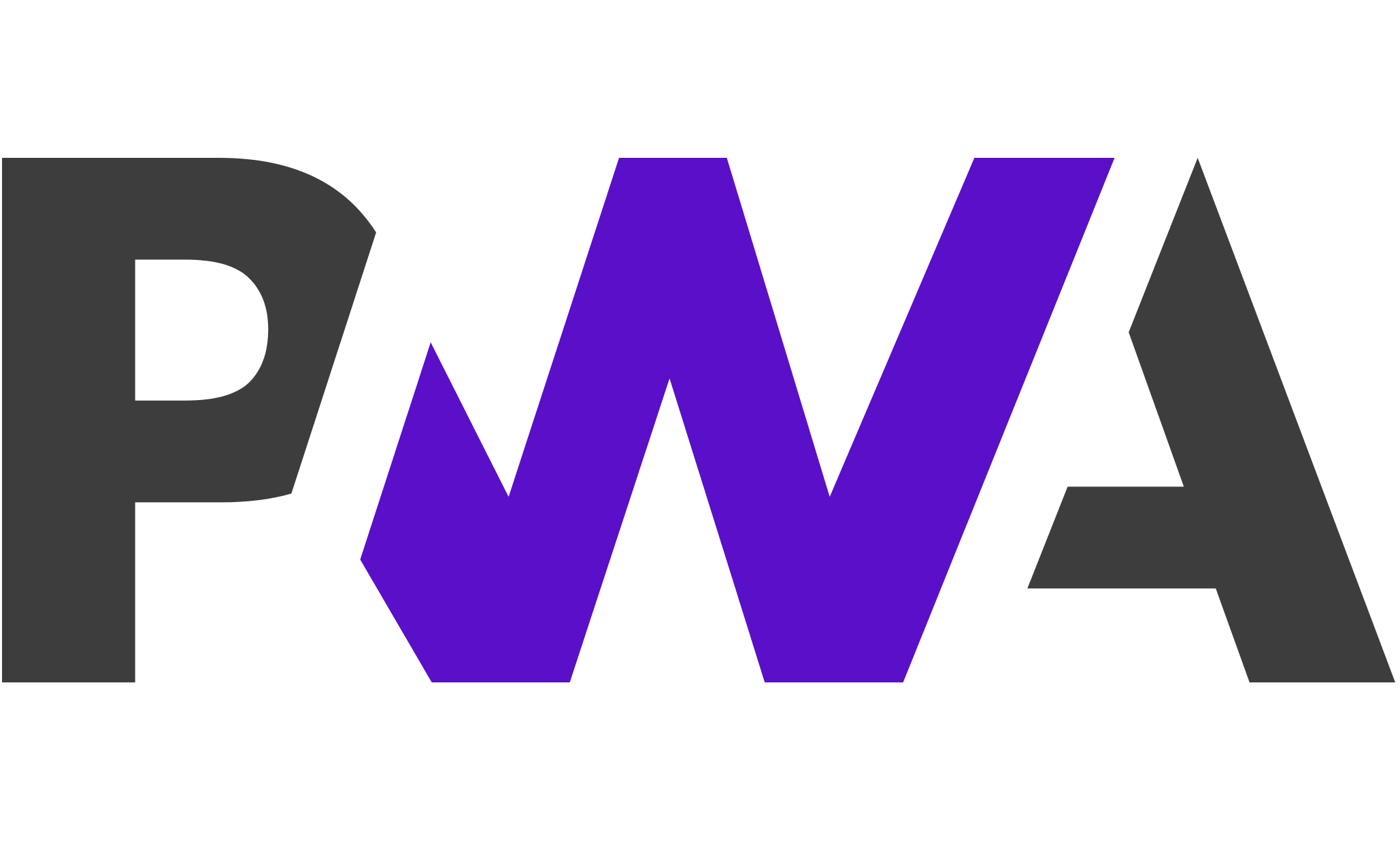
In 2015 Progressive Web Apps is introduced by Frances Berriman and Alex Russell, but before going further, what is exactly “Progressive Web Apps”?
Progressive Web Apps is “Web Applications” that taking advantage of the new features supported by modern browsers. Okay, but what is that? What is the benefit for the end users? Well, Progressive Web Apps gives the end user "reliability", so the end users will always see the content. Even if they have bad connections, they will never ever frustrated by seeing the dinosaurs page ever again. Progressive Web Apps is also engaging by giving native application feel like notification and immersive full-screen experience.
But how? There are two steps on creating Progressive Web Applications, well its not exactly two steps, you can check PWA check list on here https://developers.google.com/web/progressive-web-apps/checklist, but i will show you the big two steps to learn. The first thing is the web app manifest. What is that? It is a file that allows your application to have “native feel” like orientation, fullscreen mode, theme color, splash screen, and many more. Well, let see the example from Google Developer.
/* manifest.json */
{
"short_name": "Maps",
"name": "Google Maps",
"icons": [
{
"src": "/images/icons-192.png",
"type": "image/png",
"sizes": "192x192"
},
{
"src": "/images/icons-512.png",
"type": "image/png",
"sizes": "512x512"
}
],
"start_url": "/maps/?source=pwa",
"background_color": "#3367D6",
"display": "standalone",
"scope": "/maps/",
"theme_color": "#3367D6"
}
Well ya, its a file that makes your Progressive Web Apps feel a lot like native apps, giving an app name, icon, background color. What make its cool is, you can even make a splash screen or set your apps orientation! If you want to explore it more than i tell you, then you can visit this link https://developers.google.com/web/fundamentals/web-app-manifest/.
It's that all? No, of course not, you are missing the second step to make it feel much more like a native app, yeah it is the service worker. It is a “worker” that run a service on your web apps so it can behave like a native app, it can load your assets from the browser cache so the end users did not need to download it every time they load your web apps. Well, now you can think “oh, that makes everything make sense”. But before we learn how to add that asset to cache we have to know the service worker life cycle.
There are three steps in a service worker lifecycle, its registration, installation, and activation. To install a service worker on your web apps, you need to register it in your main Javascript code. Registration tells the browser where your service worker is located, and to start installing it in the background. So you have to register the service worker with your main js, for example:
/* main.js */
if ('serviceWorker' in navigator) {
navigator.serviceWorker.register('/sw.js')
.then(function(registration) {
console.log('Registration successful, scope is:', registration.scope);
})
.catch(function(error) {
console.log('Service worker registration failed, error:', error);
});
}
Now that you have your service worker registered on your js, then you can install your service worker in your sw.js like the example below.
/* sw.js */
const urlsToCache = [
'/',
'/url-you-want-to-cache'
];
self.addEventListener('install', function(event) {
console.log('Service worker installing...');
// I'm a new service worker
event.waitUntil(
caches.open('pages-cache-v1')
.then(cache => {
return cache.addAll(urlsToCache);
})
);
});
Then, you can activate service worker. On activate event you can remove your old cache and re-activate it if you have service worker before and want to change your caching strategy. In the example below is code that deleting cache on activate event that useful if you want to change your caching strategy.
/* sw.js */
self.addEventListener('activate', function(event) {
//perform some task
event.waitUntil(async function() {
caches.delete('pages-cache-v0.1');
}());
});
After event activate successful you are free to manipulate your web apps cache to interact with users all you want with service worker API. Now, to achieve that “native feel” we have several condition to fix right? So here is the table that we need to consider how it will be done.
Network Cache
True True
True False
False True
False False
So if the user have network and cache available we could use:
1. Stale while re-validate
This strategy is ideal for apps that frequently updating the resources where having the very latest version is non-essential, why? Because the strategy works from the pages generated and service worker will load cache and showing the cache content to the page, the service send the request after that and the requested data will be saved to the cache and will be rendered when user asking the next request.
2. Cache and network race
This strategy is ideal for small asset and slow storage access device. But if the storage access is fast that means cache will always be called first.
3. Cache then network
This strategy is rendering page from the cache first, then when the network ready, its replacing the new view from the network
4. On user interaction
Actually this behavior didn’t need cache to be present but it is a good strategy if we need to cache every user interaction like ‘Exam apps’ or like watch later on YouTube. This method triggered when use triggered an event listener like ‘click’, the network requesting data, save its data to cache
5. On network response
This kind of strategy is also use full to an apps like ‘Exam apps’. To know how this strategy works, we can start imagine if ‘Exam apps’ questions rendered to be one question one page, so whenever user answer and request the cache, its falling back to the network when the server request the next question page and update the data to cache
6. On push message → We do not implement the push notification yet
This strategy is ideal for apps that send a notification. How this strategy work? So, before the notification received, when the user receive push message the service worker intercept it, the it will request the network to get the data for the user then saving it to cache.
Then, if network is present and cache is not, we could use:
1. Network Only
This strategy is only for information that could not or should not be cached. So the service worker will not calling that resource from the cache.
2. Cache falling back to network → done
This could be happen when the cache is cleared, so the strategy is service worker asking the data from the cache first, when it does not get the cache, its getting the data or the asset from the network
But, if cache present and network is not, or in other words "offline", we could use:
1. Network Only
This strategy is only for information that could not or should not be cached. So the service worker will not calling that resource from the cache.
2. Cache falling back to network → done
This could be happen when the cache is cleared, so the strategy is service worker asking the data from the cache first, when it does not get the cache, its getting the data or the asset from the network
Last, if cache and network isnt present:
Service worker not installed, so we have base apps cache, then we couldnt do anything about it dont we?
---
Wellcode.io Team
Leading high-tech Indonesia Startup Digital - which serves the community with revolutionary products, system development, and information technology infrastructure